Table Of Content
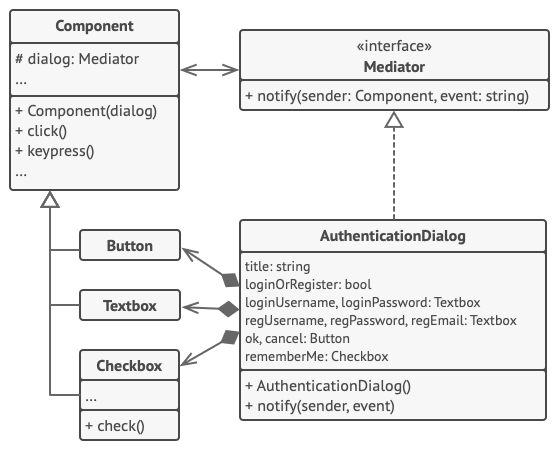
Also, in both the classes we implemented the overridden attack() and stopAttack() methods declared in the ArmedUnit interface. In the attack() method, we communicated with the mediator by calling the canAttack() method. If the method returns true, the Colleague object is confirmed that it can attack. The Mediator calls this method whenever it wants a unit to stop attacking. Programmatically we are not maintaining any reference between Colleague objects. They only communicate with the Mediator and the Mediator communicates with them.
Mediator Design Pattern in C# with Real-Time Examples
Most importantly, they don’t need to have any knowledge of one another. Thus the mediator pattern helps you write well-structured, maintainable, and easily testable code. The Mediator design pattern is a behavioral pattern that defines an object, the mediator, to centralize communication between various components or objects in a system. According to GoF definition, mediator pattern defines an object that encapsulates how a set of objects interact.
Structural code in C#
Mediator interfaces define methods of communication with components, including the notification method. This way, the Mediator pattern lets you encapsulate a complex web of relations between various objects inside a single mediator object. The fewer dependencies a class has, the easier it becomes to modify, extend or reuse that class.
Design Patterns Saga #3: Real Project Situations With Singleton
Instead, the participant only needs to check the captioning system to understand a message and then speak without knowledge of the words and their meanings used by the other attendees. In this example, considering a general scenario, the person hosting the meeting would have to learn every language and find a way to simultaneously communicate with each other. However, the Mediator pattern helps eliminate mutual dependencies between the different people on the call.
The Mediator pattern suggests that you should cease all direct communication between the components which you want to make independent of each other. Instead, these components must collaborate indirectly, by calling a special mediator object that redirects the calls to appropriate components. As a result, the components depend only on a single mediator class instead of being coupled to dozens of their colleagues. In the programming world, you can easily model this requirement by creating classes for each armed unit. In each class, whenever its object is about to start attacking, you can implement the logic to notify objects of the other classes.
Let’s write some test code so we can observe the Mediator Pattern in action. The participants in this design are the mediator, the concrete mediator, and one or more participant types. Note that the participant type is sometimes called a colleague.
Some other Popular Design Patterns
In the mediator design pattern, the objects don’t communicate with one another directly but through the mediator. When an object needs to communicate with another object or a set of objects, it transmits the message to the mediator. The mediator then transmits the message to each receiver object in a form that is understandable to it. Mediator is a behavioral design pattern that lets you reduce chaotic dependencies between objects. The pattern restricts direct communications between the objects and forces them to collaborate only via a mediator object. The Mediator pattern is considered one of the most important and widely adopted patterns.
The Chatroom is the central hub through which all communication takes place. At this point only one-to-one communication is implemented in the Chatroom, but would be trivial to change to one-to-many. By implementing this logic directly in the form element classes, it becomes much harder to reuse these classes in other forms. The users within the chatroom won’t talk to each other directly. Instead, the chatroom serves as the mediator between the users. You can compare this pattern to the relationship between an air traffic controller and a pilot.
A pattern-based approach to protocol mediation for web services composition - ScienceDirect.com
A pattern-based approach to protocol mediation for web services composition.
Posted: Thu, 28 Dec 2017 11:27:51 GMT [source]
The system objects that communicate each other are called Colleagues. Usually we have an interface or abstract class that provides the contract for communication and then we have concrete implementation of mediators. For our example, we will try to implement a chat application where users can do group chat. Every user will be identified by it’s name and they can send and receive messages.
Create a class file named ConcreteUser.cs and copy and paste the following code. This is a concrete class that implements the User abstract class and provides the implementation for the Send and Receive abstract methods. The Send method sends a message to a particular Facebook group.
It makes the communication between objects encapsulated with a mediator object. Objects don’t communicate directly with each other, but instead, they communicate through the mediator. The essence of the mediator pattern is to "define an object that encapsulates how a set of objects interact". If the objects interact directly with one other, the system components will be strongly connected with each other, resulting in increased maintainability costs and ease of extension. The Mediator pattern focuses on acting as a bridge between items to facilitate communication and aid in the implementation of lose-coupling between components.
By implementing this pattern, we can create a bidirectional communication layer, which can be used by a class in order to interact with others through a common object acting as a mediator. With the mediator pattern, communication between objects is encapsulated within a mediator object. Objects no longer communicate directly with each other, but instead communicate through the mediator. This reduces the dependencies between communicating objects, thereby reducing coupling.
So, create an abstract class named User.cs and copy and paste the following code. This abstract class has two abstract methods, i.e., Send and Receive. The pattern lets you extract all the relationships between classes into a separate class, isolating any changes to a specific component from the rest of the components.
In the worst case, imagine that the battle tactics change so that both the soldier and sniper units can now attack simultaneously. We can address such challenges, similarly as done in real life. Place a Commander in a base camp that will act as the mediator.
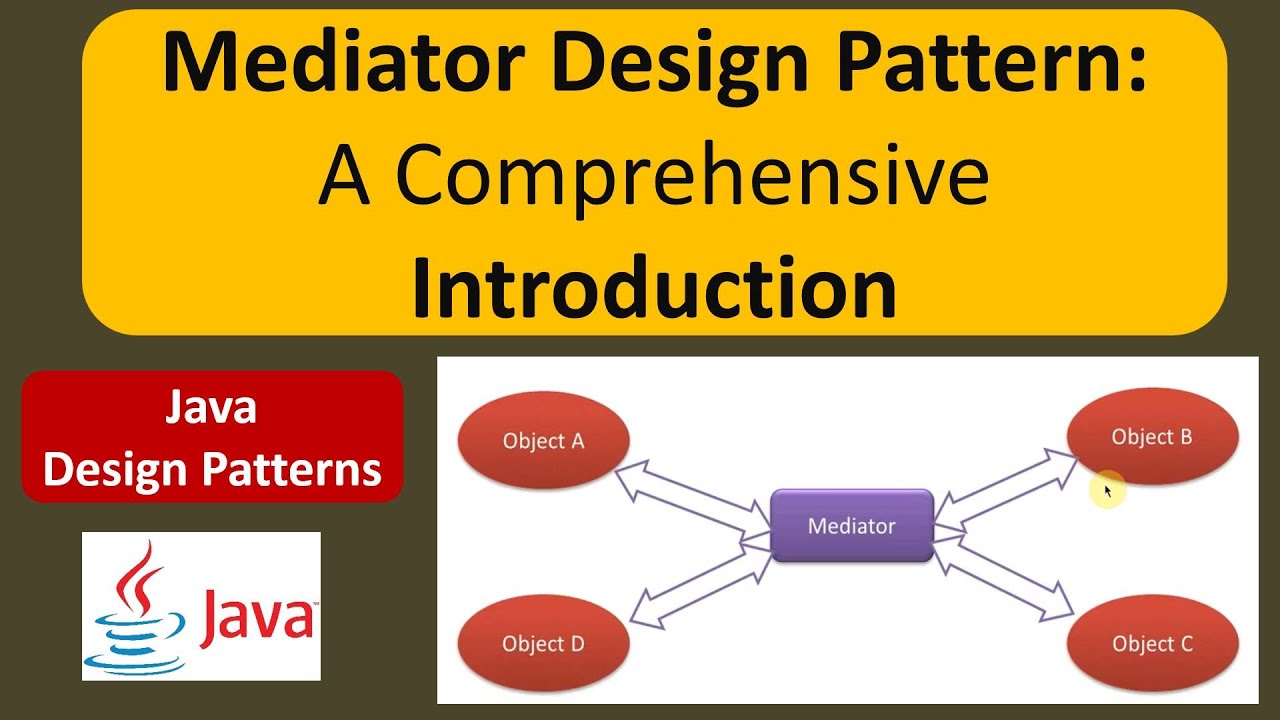
The sender doesn’t know who will end up handling the request, and the receiver doesn’t know who sent it in the first place. Each talks tothe Mediator, which in turn knows and conducts the orchestration ofthe others. The "many to many" mapping between colleagues that wouldotherwise exist, has been "promoted to full object status". This newabstraction provides a locus of indirection where additional leveragecan be hosted. Although the different participants on the call seem to be communicating directly, they don't.
Instead, they speak to an air traffic controller, who sits in a tall tower somewhere near the airstrip. Without the air traffic controller, pilots would need to be aware of every plane in the vicinity of the airport, discussing landing priorities with a committee of dozens of other pilots. Misuse of a mediator can result in crippling the interfaces of the mediator's colleague classes. We will next move ahead and create the Colleague interface and the implementation classes.